What are the uses of such a hook? Maybe adding control information to the file or perhaps to manage image names. The possibilities are endless.
How the Translate Process Works
Various Novaworks applications allow for the translation of data from applications such as Microsoft Word or Excel. The process is invoked by the user by opening a file, importing to a new file, or importing into an existing file. During each of these actions the application’s translate module comes into play to aid in the selection of an appropriate conversion method, options, and destination. Setting a post process hook allows the data to be intercepted prior to being inserted in to the destination window or file.
When the hook is run, it receives the parameters: source, destination, what method was used, and any related options. If our hook is not interested in the file, it can just ignore the operation. For example, let’s say that we want to post process an HTML conversion but the user instead runs Word to text conversion. The hook can simply exit and do nothing.
Setting the Hook
To set the hook, the TranslateSetPostProcessHook function is used. This can be called anywhere but would generally be set when the application starts up. The function parameters are as follows:
int = TranslateSetPostProcessHook ( string script, string function );
The script parameter is the name of the script to run. If the string is empty, the name and location of the currently executing script is used. The function parameter specifies the function to run after conversion has completed.
The Hook
After a conversion process has completed, the hook is called before the data is placed into the destination window or file. The hook takes the following form:
int post_process_hook (string xl_type,
string xl_src, string xl_dst, string xl_dst_base,
dword xl_flags, string xl_options );
The xl_type parameter will contain the name of the conversion method used. For example, the value could be ‘DOCtoHTML’ for a conversion from Microsoft Word. Based on this parameter, the post process can run different operations or simply ignore the conversion completely.
The xl_src parameter is the name of the source file. This is provided for reference or if the script wants to examine the content. Normally, data is post processed using the destination file.
The xl_dst parameter is the name of the destination file. This is the file that the post process script modifies.
The xl_dst_base parameter specifies the location of the destination. Depending on the context, the destination file may be located in the Windows temp folder. This path can be used reference images and other resources.
Flags are provided as a dword in the xl_flags parameter to direct operation. For example, if the XL_QUIET bit is set, then the script should not interact with the user.
Finally, the xl_options string contains ‘property: value’ pairs of information for settings.
The parameter names can be named at the programmer’s convenience but the datatype must match. When the script completes its processing, it should return ERROR_NONE. Returning an error will cause the import process to terminate.
General Operation
For HTML files, as a programmer you can either use the SGML mini parse functions or the SGML Object. The latter, while more complex, certainly allows for more options for processing. For example, an object can be created using the SGMLCreate function, by attaching a file by using the SGMLSetFile function, or by attaching a Mapped Text Object. The file can be scanned, changes can be made, and then by using the SGMLWriteTag function, the file can be updated. At the end of the process, the destination file can be saved and the importing will complete upon exiting the hook.
Here is an example to add information to the log and inject data into a “TexttoHTML” conversion:
int main() {
int rc;
rc = TranslateSetPostProcessHook("", "post_translate");
if (IsError(rc)) {
MessageBox('x', "Error %08X setting the hook.", rc);
return rc;
}
MessageBox('i', "Hookset for 'TexttoHTML'");
return ERROR_NONE;
}
int post_translate(string type, string fnSource, string fnFile,
string fnPath, dword flags, string options) {
string s1;
int rc,
cx;
AddMessage("Add Some Stuff to the Log:");
LogIndent();
LogSetMessageType(LOG_ERROR);
AddMessage("Force a log error to for the log to display");
LogSetMessageType(LOG_INFO);
AddMessage("Type: %s", type);
AddMessage("fnSource: %s", fnSource);
AddMessage("fnFile: %s", fnFile);
AddMessage("fnPath: %s", fnPath);
AddMessage("flags: %08X", flags);
AddMessage("Options: %s", options);
if (type == "TexttoHTML") {
s1 = FileToString(fnFile);
if (s1 == "") {
AddMessage("Error processing data: %08X (opening file)", GetLastError());
return ERROR_NONE;
}
cx = FindInString(s1, "<BODY>");
if (cx < 0) { cx = 0; }
else { cx += 6; }
s1 = InsertStringSegment(s1, "\r\n\r\n<P STYLE='text-align: center; color: red'><B>POST PROCESS SUCCESS</B></P>", cx);
rc = StringToFile(s1, fnFile);
if (IsError(rc)) {
AddMessage("Error writing data: %08X (saving file)", rc);
return ERROR_NONE;
}
}
return ERROR_NONE;
}
In the above case, once the script is run from the IDE or as part of startup, the translate operation becomes hooked. Once that’s accomplished, the post_translate function will be run at the end of every translate operation. This script adds some information to the log before checking the filter. On a match, the function injects some HTML into the file just to illustrate how the destination can be modified.
As a side note, if you copy and paste the above script into the Legato IDE, you must save it to allow the hook to be accessed. As with all hooks the program requires the script file to be named. Otherwise, the TranslateSetPostProcessHook function will fail.
On importing a text file, select the “TexttoHTML” destination:
When the translation operation is completed, the file will have the following added:
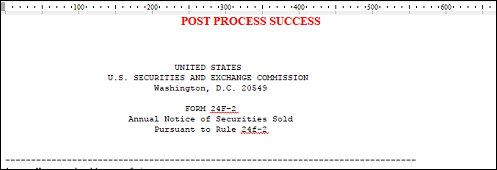
With log entries as follows:
Basically, the hook has full control over the contents of the destination file. The program can use the SGML parser to make HTML changes, contact external resources, or essentially run any file level function. Since the file is not yet a window, edit-level menu type functions cannot be run by functions such as RunMenuFunction.
Finally
Your post process script can also have options implemented using the settings functions. User access can be added using an extension tool via the MenuAddFunction function.
Here are some additional ideas for useful post-process scripting:
— Processing image names and renaming them to match a specific project or job number.
— Adding document tracking information.
— Running functions on tables, list, fields, drop caps, etc...
Keep in mind that as a programmer, you also can define and add new translate modules as you see fit using the TranslateAddHook function. While this is the ultimate power in conversion, it requires you to do all the heavy lifting. On the other hand, the post process translate hook gives you the opportunity to customize code and control the processing of newly converted data by building on existing translation functions.
If you have any questions, feel free to contact technical support.
Scott Theis is the President of Novaworks and the principal developer of the Legato scripting language. He has extensive expertise with EDGAR, HTML, XBRL, and other programming languages. |
Additional Resources
Novaworks’ Legato Resources
Legato Script Developers LinkedIn Group
Primer: An Introduction to Legato