While writing Legato scripts, a common task is to alert a user or list of users about an action taking place. One of the best ways to do this is through sending an email. Legato has a very handy built-in function EmailSendMessage, that can do this for you with very little effort. Below is a basic script that shows how you can send an email.
//Tests if your email is set up, and if so, emails a basic message
string message;
string to;
string subject;
int rc;
if (IsEmailSMTPSetup()){
to = "support@novaworkssoftware.com";
subject = "My Test Email";
message = "<P>The email can have HTML in it.</P>";
rc = EmailSendMessage(to,subject,message);
if (IsError(rc)){
MessageBox('x',"Cannot send email. Error: %0x",rc);
}
else{
MessageBox('i',"Email sent successfully.");
}
}
else{
MessageBox('x',"Please Setup your email first.");
}
The SendEmailMessage function requires 3 parameters: to, subject, and body. Each
parameter is a string. The to parameter contains the email addresses that are going to receive this message. You can specify
multiple email addresses in this string if they are separated by semicolons. The subject string contains the subject of the email.
Any content in this string can effect junk mail rating, so be careful when choosing what to put here. Finally, the body
string has the entire content of the message. You can include HTML in the body or it can be plain text. Attachments can be added
to the body, but they must be encoded manually. Legato doesn’t have functions to automate adding of attachments at this
point.
In order to send an email with Legato, you must have GoFiler set
up to actually use SMTP email. You can find the email settings in GoFiler under File->Preferences, under the Services
tab. Just select SMTP for mail and enter the required server information. It’s the same information you would need if you
were setting up an email client like Thunderbird or Outlook. The sent email isn’t encrypted, so make sure that you do
not use this function to send confidential information.
The Preferences Screen for Editing SMTP Settings:
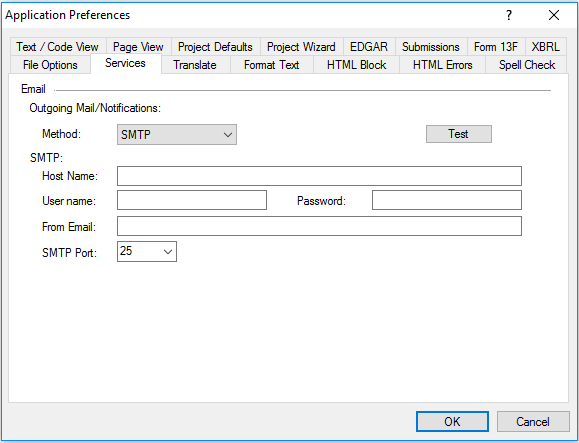
When using the SendEmailMessage function to send email, it’s
important to check the return code of the function to ensure that it was sent successfully. If the return code is ERROR_NONE
(0), it means that the message was posted for sending without error. If any other error results, it could mean a lot of other
things may have possibly gone wrong, for example, the SMTP settings on Gofiler being incorrect, the password being incorrect,
or the mail server was unreachable. Note that a successful return code doesn’t mean the
message was delivered successfully. It only means that Legato’s part in sending the message returned without error. There
could still be server issues with sending the message.
This function can be used in combination with other Legato functions to create a huge
variety of functions. For example, you could use an ODBC connector (covered in previous blog posts) to pull database
information and use Legato’s HTML Writer Object (see the Legato SDK for more information on this) to format the data
into a nice HTML document. You could also use Legato’s collaboration functions to build task lists and notifications
and then email users these lists to keep track of how projects are progressing. Sending email is a basic way to notify users
of changing data or any other information they may need, and Legato provides a simple way to do so.
Steven Horowitz has been working for Novaworks for over five years as a technical expert with a focus on EDGAR HTML and XBRL. Since the creation of the Legato language in 2015, Steven has been developing scripts to improve the GoFiler user experience. He is currently working toward a Bachelor of Sciences in Software Engineering at RIT and MCC. |
Additional Resources
Novaworks’ Legato Resources
Legato Script Developers LinkedIn Group
Primer: An Introduction to Legato